Understanding Object-Oriented Programming in C++
Understanding Object-Oriented Programming in C++
Introduction: Object-oriented programming (OOP) is a powerful paradigm that revolutionized the way we write software. With its emphasis on encapsulation, inheritance, and polymorphism, OOP enables developers to create modular, reusable, and maintainable code. In this blog post, we will explore the fundamentals of OOP in C++, one of the most popular programming languages for building robust and efficient applications.
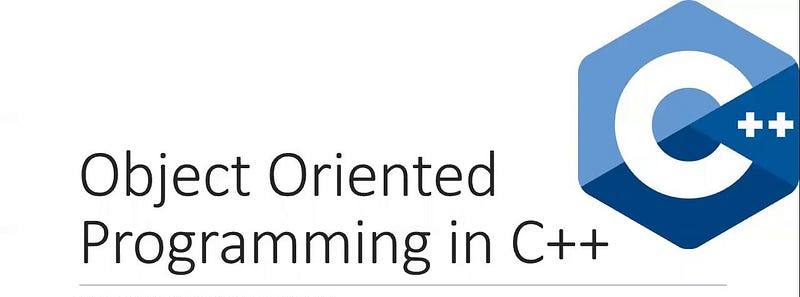
- Objects and Classes: At the heart of OOP in C++ are objects and classes. An object is a real-world entity with its own state and behavior, while a class serves as a blueprint for creating objects. In C++, classes are defined using the
class
keyword and can contain data members (variables) and member functions (methods). By instantiating a class, we create an object that possesses the properties and behaviors defined within the class. - Encapsulation: Encapsulation is the principle of bundling data and methods within a class, hiding the internal implementation details from the outside world. In C++, this is achieved through access specifiers:
public
,private
, andprotected
. Public members are accessible from any part of the program, private members are only accessible within the class itself, and protected members are accessible within the class and its derived classes. Encapsulation helps in achieving data abstraction, data security, and code reusability. - Inheritance: Inheritance allows us to create new classes (derived classes) from existing ones (base classes). The derived class inherits the data members and member functions of the base class, enabling code reuse and specialization. In C++, inheritance is implemented using the
class
keyword followed by a colon and the access specifier (public
,private
, orprotected
) along with the base class name. Inheritance promotes code extensibility, hierarchy, and modularity. - Polymorphism: Polymorphism, derived from the Greek words “poly” (many) and “morphos” (forms), allows objects of different classes to be treated as objects of a common base class. Polymorphism can be achieved in C++ through function overloading and function overriding. Function overloading enables multiple functions with the same name but different parameters, while function overriding occurs when a derived class provides its own implementation of a base class’s method. Polymorphism promotes code flexibility, extensibility, and modifiability.
- Abstraction: Abstraction is the process of simplifying complex systems by breaking them down into smaller, more manageable parts. In C++, abstraction can be achieved through classes and objects. By hiding unnecessary details and exposing only the essential features, we create abstract data types (ADTs) that facilitate code organization and promote high-level thinking.
Conclusion: Object-oriented programming is a fundamental concept that has transformed the way we design and develop software. C++ provides robust support for OOP principles, including encapsulation, inheritance, polymorphism, and abstraction. By leveraging these concepts, developers can create modular, maintainable, and scalable code, leading to more efficient software development. Whether you’re a beginner or an experienced programmer, understanding and mastering OOP in C++ will undoubtedly enhance your skills and enable you to build powerful applications. So dive in, experiment, and embrace the world of OOP in C++!
Remember, practice makes perfect, so start coding and exploring the vast possibilities of OOP in C++. Happy coding!
Comments
Post a Comment