Callback function in JavaScript
Callback function in JavaScript
What is a callback function in JavaScript?
A callback function is a function that is passed as an argument to another function and is executed after the first function has completed its task. Callback functions are useful for handling asynchronous events, such as network requests, file operations, or timers. For example, you can use a callback function to display the result of a calculation after the calculation is done.
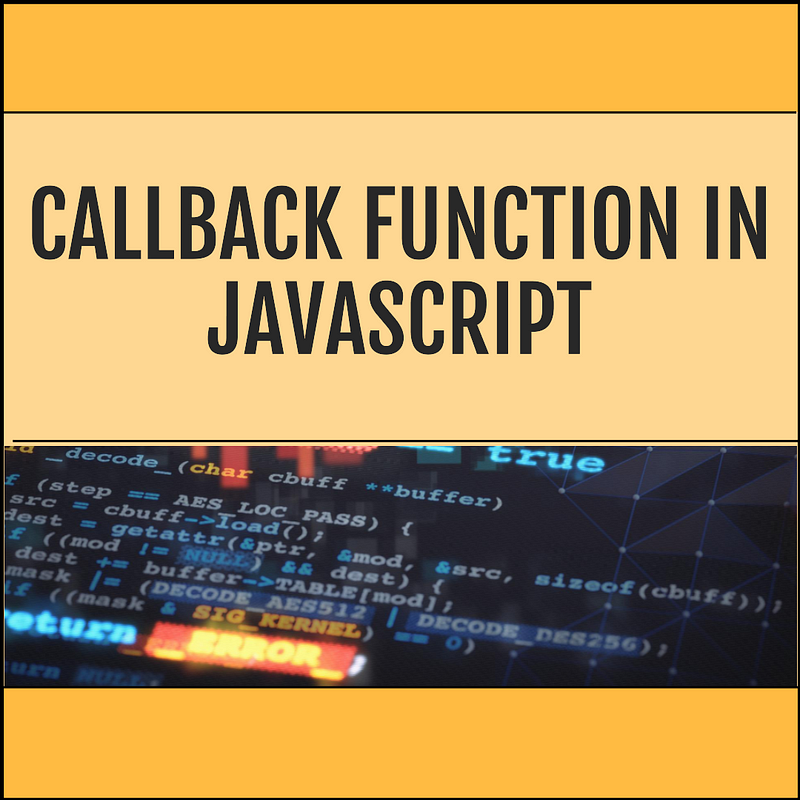
Here is a simple example of a callback function in JavaScript:
// Define a function that takes a callback as an argument
function sayHello(callback) {
// Do some task
console.log("Hello, world!");
// Invoke the callback function
callback();
}
// Define another function
function sayGoodbye() {
// Do some task
console.log("Goodbye, world!");
}
// Call the first function and pass the second function as a callback
sayHello(sayGoodbye);
// The output will be:
// Hello, world!
// Goodbye, world!
How do I pass arguments to a callback function?
There are different ways to pass arguments to a callback function in JavaScript. One way is to use an anonymous function as a wrapper for the callback function and pass the arguments inside the wrapper. For example:
// Define a callback function that takes two arguments
function tryMe(param1, param2) {
console.log(param1 + " and " + param2);
}
// Define a function that takes a callback as an argument
function callbackTester(callback) {
// Do some task
console.log("Testing callback");
// Invoke the callback function
callback();
}
// Call the tester function and pass an anonymous function as a callback
callbackTester(function() {
// Pass the arguments to the callback function inside the anonymous function
tryMe("hello", "goodbye");
});
// The output will be:
// Testing callback
// hello and goodbye
Break into the Code:
This code is an example of using a callback function in JavaScript. A callback function is a function that is passed as an argument to another function and is executed after the first function has completed its task. Here is a line-by-line explanation of the code:
- Line 1: Define a callback function named
tryMe
that takes two arguments,param1
andparam2
. - Line 2: Inside the callback function, use the
console.log
method to print the values ofparam1
andparam2
with the word “and” in between. - Line 3: Close the callback function with a curly brace.
- Line 5: Define another function named
callbackTester
that takes a callback function as an argument, namedcallback
. - Line 6: Inside the tester function, use the
console.log
method to print the message “Testing callback”. - Line 8: Invoke the callback function by calling it with parentheses.
- Line 9: Close the tester function with a curly brace.
- Line 11: Call the tester function and pass an anonymous function as a callback argument. An anonymous function is a function that has no name and is defined on the spot.
- Line 12: Inside the anonymous function, pass the arguments “hello” and “goodbye” to the callback function
tryMe
by calling it with parentheses. - Line 13: Close the anonymous function with a curly brace and a semicolon.
- Line 15: The output of the code will be the following messages printed in the console:
- Testing callback
- hello and goodbye
This code demonstrates how a callback function can be used to perform some task after another function has finished its work. It also shows how to pass arguments to a callback function using an anonymous function or the bind
method. You can learn more about callback functions from this part of the current page.
Using bind()
Another way is to use the bind()
method to create a new function with the arguments bound to the callback function. For example:
// Define a callback function that takes two arguments
function tryMe(param1, param2) {
console.log(param1 + " and " + param2);
}
// Define a function that takes a callback as an argument
function callbackTester(callback) {
// Do some task
console.log("Testing callback");
// Invoke the callback function
callback();
}
// Call the tester function and pass a bound function as a callback
callbackTester(tryMe.bind(null, "hello", "goodbye"));
// The output will be:
// Testing callback
// hello and goodbye
Break into the Code:
This code is an example of using a callback function in JavaScript. A callback function is a function that is passed as an argument to another function and is executed after the first function has completed its task. Here is a line-by-line explanation of the code:
- Line 1: Define a callback function named
tryMe
that takes two parameters,param1
andparam2
. - Line 2: Use the
console.log
method to print the values ofparam1
andparam2
with the word “and” in between. - Line 3: Close the function definition with a semicolon.
- Line 5: Define another function named
callbackTester
that takes one parameter,callback
. - Line 6: Use the
console.log
method to print the message “Testing callback”. - Line 8: Invoke the
callback
function that was passed as an argument tocallbackTester
. - Line 9: Close the function definition with a semicolon.
- Line 11: Call the
callbackTester
function and pass a bound function as a callback. A bound function is a new function that is created by using thebind
method on an existing function and specifying some arguments to be fixed. In this case, the bound function is created by using thebind
method on thetryMe
function and passingnull
as the first argument and “hello” and “goodbye” as the second and third arguments. Thenull
argument means that thethis
value of the bound function will be the same as the original function. The “hello” and “goodbye” arguments mean that theparam1
andparam2
parameters of the bound function will be fixed to those values. - Line 13: The output of the code will be “Testing callback” followed by “hello and goodbye”. This is because the
callbackTester
function prints “Testing callback” and then calls the bound function, which prints “hello and goodbye”.
Can you give me an example of using callbacks in real-world applications?
Sure, I can give you some examples of using callbacks in real-world applications. Callbacks are functions that are passed as arguments to other functions and are executed after the other functions have completed their tasks. Callbacks are useful for handling asynchronous events, such as network requests, file operations, or timers.
Here are some common scenarios where callbacks are used:
- Event handling: If you’re working with client-side JavaScript, you’ll often use event listeners to handle user interactions, such as clicking a button, typing in a text field, or moving the mouse. Event listeners are functions that are registered with an event source, such as an HTML element, and are invoked when the event occurs. For example, you can use a callback function to display a message when a button is clicked:
// Get the button element by its id
var button = document.getElementById("myButton");
// Define a callback function that will be executed when the button is clicked
function showMessage() {
alert("You clicked the button!");
}
// Add an event listener to the button and pass the callback function as an argument
button.addEventListener("click", showMessage);
- Unit testing: If you’ve done any JavaScript unit testing, you’ve likely seen callbacks here as well. Unit testing frameworks, such as Mocha or Jasmine, use callbacks to define test cases and assertions. For example, you can use a callback function to test the functionality of a calculator module:
// Import the calculator module
var calculator = require("./calculator");
// Define a test suite using a callback function
describe("Calculator", function() {
// Define a test case using another callback function
it("should add two numbers", function() {
// Use an assertion library, such as Chai, to verify the expected result
expect(calculator.add(2, 3)).to.equal(5);
});
});
- Asynchronous operations: In web programming, a lot of things we need to do are asynchronous, meaning that they don’t block the execution of the code until they are finished. For example, when we make a network request to fetch some data from a server, we don’t want to wait until the response arrives before we can do anything else. Instead, we want to continue with other tasks and handle the response when it’s ready. To do this, we use callbacks to specify what to do with the response data. For example, you can use a callback function to display the data from a JSON file:
// Create a new XMLHttpRequest object
var xhr = new XMLHttpRequest();
// Define a callback function that will be executed when the request is done
function handleResponse() {
// Check if the request was successful
if (xhr.status === 200) {
// Parse the response data as JSON
var data = JSON.parse(xhr.responseText);
// Display the data in the console
console.log(data);
} else {
// Display an error message
console.error("Request failed: " + xhr.status);
}
}
// Open the request with the GET method and the URL of the JSON file
xhr.open("GET", "https://example.com/data.json");
// Register the callback function with the onload event
xhr.onload = handleResponse;
// Send the request
xhr.send();
I hope these examples helped you understand how callbacks work in JavaScript. You can learn more about callback functions from these sources:
- JavaScript Callbacks — W3Schools
- Callback function — MDN Web Docs
- A Guide to Callback Functions in JavaScript | Built In
- JavaScript | Callbacks | Codecademy
- What is a Callback Function in JavaScript? JS Callbacks Example Tutorial
- JavaScript Callbacks — W3Schools
- Callback function — MDN Web Docs
- A Guide to Callback Functions in JavaScript | Built In
- JavaScript | Callbacks | Codecademy
- What is a Callback Function in JavaScript? JS Callbacks Example Tutorial
- JavaScript: Passing parameters to a callback function — Stack Overflow
- Passing arguments to callback functions — jstips.co
- JavaScript Passing parameters to a callback function — GeeksforGeeks
Comments
CustomerID INT PRIMARY KEY,
CustomerName VARCHAR(255) NOT NULL,
ContactName VARCHAR(255) NOT NULL,
Address VARCHAR(255) NOT NULL,
City VARCHAR(255) NOT NULL,
PostalCode VARCHAR(10) NOT NULL,
Country VARCHAR(255) NOT NULL
);
Post a Comment