Bitwise Operators in C/C++
Bitwise Operators in C/C++
In C, the following 6 operators are bitwise operators (also known as bit operators as they work at the bit-level). They are used to perform bitwise operations in C.
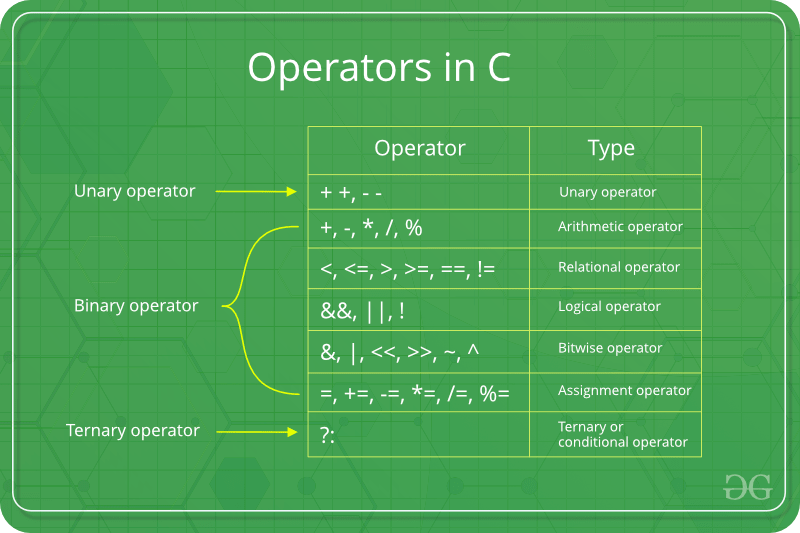
The & (bitwise AND) in C or C++ takes two numbers as operands and does AND on every bit of two numbers. The result of AND is 1 only if both bits are 1.
The | (bitwise OR) in C or C++ takes two numbers as operands and does OR on every bit of two numbers. The result of OR is 1 if any of the two bits is 1.
The ^ (bitwise XOR) in C or C++ takes two numbers as operands and does XOR on every bit of two numbers. The result of XOR is 1 if the two bits are different.
The << (left shift) in C or C++ takes two numbers, the left shifts the bits of the first operand, and the second operand decides the number of places to shift.
The >> (right shift) in C or C++ takes two numbers, right shifts the bits of the first operand, and the second operand decides the number of places to shift.
The ~ (bitwise NOT) in C or C++ takes one number and inverts all bits of it.
Let’s look at the truth table of the bitwise operators.
X | Y | X & Y | X | Y | X ^ Y |
---|---|---|---|---|
0 | 0 | 0 | 0 | 0 |
0 | 1 | 0 | 1 | 1 |
1 | 0 | 0 | 1 | 1 |
1 | 1 | 1 | 1 | 0 |
Comments
#include
int main(){
int bt[10], at[10], wt[10], tat[10], n;
printf("Enter process no: ");
scanf("%d", &n);
for(int i=0; i<n; i++){
printf("Enter burst time of process: ");
scanf("%d", &bt[i]);
}
float awt=0, atat=0;
wt[0] = 0;
tat[0] = bt[0] + wt[0];
atat += tat[0];
for(int i=1; i<n; i++){
wt[i] = bt[i-1] + wt[i-1];
awt += wt[i];
tat[i] = bt[i] + wt[i];
atat += tat[i];
}
atat /= n;
awt /= n;
printf("Process\t\tBT\tWT\tTAT\t\n");
for(int i=0; i<n; i++){
printf("p[%d]\t\t%d\t%d\t%d\n", i+1, bt[i], wt[i], tat[i]);
}
printf("Avg wt: %f\nAvg tat: %f\n", awt, atat);
}
#include
int main()
{
int i, n, j, temp;
int bt[100], wt[100], tat[100], p[100];
float awt = 0, atat = 0;
printf("Shortest Job First Scheduling Non-Primitive(Simultaneous Arrival)\n");
printf("Enter the No. of processes : ");
scanf("%d", &n);
for (i = 0; i < n; i++)
{
// printf("Enter the arrival time of %d process :\n",i+1);
// scanf("%d",&at[i]);
printf("Enter the burst time of %d process : ", i + 1);
scanf("%d", &bt[i]);
p[i] = i + 1;
}
/*Sorting According to Burst Time*/
for (i = 0; i < n; i++)
{
for (j = i + 1; j < n; j++)
{
if (bt[i] > bt[j])
{
temp = bt[j];
bt[j] = bt[i];
bt[i] = temp;
temp = p[j];
p[j] = p[i];
p[i] = temp;
}
}
}
/*waiting time & turnaround time calculation of every process*/
wt[0] = 0;
tat[0] = bt[0] + wt[0];
atat += tat[0];
for (i = 1; i < n; i++)
{
wt[i] = bt[i - 1] + wt[i - 1];
awt += wt[i];
tat[i] = wt[i] + bt[i];
atat += tat[i];
}
atat /= n;
awt /= n;
printf("Process.\tB.T.\tW.T.\tT.A.T.\n");
for (i = 0; i < n; i++)
{
printf("P[%d]\t\t%d\t%d\t%d\n", p[i], bt[i], wt[i], tat[i]);
}
printf("Average Waiting Time: %0.3f\nAverage Turn Around Time:%0.3f", awt, atat);
return 0;
}
#include
int main()
{
int bt[20], p[20], wt[20], tat[20], pr[20], i, j, n, total = 0, pos, temp;
float awt = 0, atat = 0;
printf("Enter Total Number of Process:");
scanf("%d", &n);
printf("\nEnter Burst Time and Priority\n");
for (i = 0; i < n; i++)
{
printf("\nP[%d]\n", i + 1);
printf("Burst Time:");
scanf("%d", &bt[i]);
printf("Priority:");
scanf("%d", &pr[i]);
p[i] = i + 1; // contains process number
}
// sorting burst time, priority and process number in ascending order using selection sort
for (i = 0; i < n; i++)
{
for (j = i + 1; j < n; j++)
{
if (pr[j] < pr[i])
{
temp = pr[i];
pr[i] = pr[j];
pr[j] = temp;
temp = bt[i];
bt[i] = bt[j];
bt[j] = temp;
temp = p[i];
p[i] = p[j];
p[j] = temp;
}
}
}
/*waiting time & turnaround time calculation of every process*/
wt[0] = 0;
tat[0] = bt[0] + wt[0];
atat += tat[0];
for (i = 1; i < n; i++)
{
wt[i] = bt[i - 1] + wt[i - 1];
awt += wt[i];
tat[i] = wt[i] + bt[i];
atat += tat[i];
}
atat /= n;
awt /= n;
printf("\nProcess\t Burst Time \tWaiting Time\tTurnaround Time");
for (i = 0; i < n; i++)
{
tat[i] = bt[i] + wt[i]; // calculate turnaround time
total += tat[i];
printf("\nP[%d]\t\t %d\t\t %d\t\t\t%d", p[i], bt[i], wt[i], tat[i]);
}
printf("\n\nAverage Waiting Time=%d", awt);
printf("\nAverage Turnaround Time=%d\n", atat);
return 0;
}
#include
int main(){
int bt[100], tq, i, n, rembt[100], t = 0, wt[100], c = 0, p[100], tat[100];
double total = 0, avgwt = 0;
printf("Enter process number: ");
scanf("%d", &n);
for (i = 0; i < n; i++){
printf("Input burst time for process P%d\n", i + 1);
scanf("%d", &bt[i]);
p[i] = i + 1;
}
printf("Take input time quantum.\n");
scanf("%d", &tq);
for (i = 0; i < n;i++){
rembt[i] = bt[i];
}
while (c!=n)
{
for (i = 0; i < n; i++){
if(rembt[i]>tq){
t += tq;
rembt[i] -= tq;
}else if(rembt[i]!=0){
t += rembt[i];
wt[i] = t - bt[i];
tat[i] = t;
total += wt[i];
rembt[i] = 0;
c++;
}
}
}
avgwt = total / n;
printf("Process\t\tBT\tWT\tTAT\n");
for (i = 0; i < n; i++){
total == bt[i];
printf("P[%d]\t\t%d\t%d\t%d\n", p[i], bt[i], wt[i], tat[i]);
}
double avgtt = total / n;
printf("Average waiting time: %f\n", avgwt);
printf("Average turnaround time: %f\n", avgtt);
return 0;
}
#include
void quicksort(int number[10],int first,int last)
{
int i, j, pivot, temp;
if(firstnumber[pivot])
j--;
if(i<j)
{
temp=number[i];
number[i]=number[j];
number[j]=temp;
}
}
temp=number[pivot];
number[pivot]=number[j];
number[j]=temp;
quicksort(number,first,j-1);
quicksort(number,j+1,last);
}
}
int main()
{
int i, count, number[10];
printf("Enter some elements (Max. - 10): ");
scanf("%d",&count);
printf("Enter %d elements: ", count);
for(i=0; i<count; i++)
scanf("%d",&number[i]);
quicksort(number,0,count-1);
printf("The Sorted Order is: ");
for(i=0; i<count; i++)
printf(" %d",number[i]);
return 0;
}
// Max-Heap data structure in C
#include
int size = 0;
void swap(int *a, int *b)
{
int temp = *b;
*b = *a;
*a = temp;
}
void heapify(int array[], int size, int i)
{
if (size == 1)
{
printf("Single element in the heap");
}
else
{
int largest = i;
int l = 2 * i + 1;
int r = 2 * i + 2;
if (l < size && array[l] > array[largest])
largest = l;
if (r < size && array[r] > array[largest])
largest = r;
if (largest != i)
{
swap(&array[i], &array[largest]);
heapify(array, size, largest);
}
}
}
void insert(int array[], int newNum)
{
if (size == 0)
{
array[0] = newNum;
size += 1;
}
else
{
array[size] = newNum;
size += 1;
for (int i = size / 2 - 1; i >= 0; i--)
{
heapify(array, size, i);
}
}
}
void deleteRoot(int array[], int num)
{
int i;
for (i = 0; i < size; i++)
{
if (num == array[i])
break;
}
swap(&array[i], &array[size - 1]);
size -= 1;
for (int i = size / 2 - 1; i >= 0; i--)
{
heapify(array, size, i);
}
}
void printArray(int array[], int size)
{
for (int i = 0; i < size; ++i)
printf("%d ", array[i]);
printf("\n");
}
int main()
{
int array[10];
insert(array, 3);
insert(array, 4);
insert(array, 9);
insert(array, 5);
insert(array, 2);
printf("Max-Heap array: ");
printArray(array, size);
deleteRoot(array, 4);
printf("After deleting an element: ");
printArray(array, size);
}
#include
#define max 10
int a[11] = { 10, 14, 19, 26, 27, 31, 33, 35, 42, 44, 0 };
int b[10];
void merging(int low, int mid, int high) {
int l1, l2, i;
for(l1 = low, l2 = mid + 1, i = low; l1 <= mid && l2 <= high; i++) {
if(a[l1] <= a[l2])
b[i] = a[l1++];
else
b[i] = a[l2++];
}
while(l1 <= mid)
b[i++] = a[l1++];
while(l2 <= high)
b[i++] = a[l2++];
for(i = low; i <= high; i++)
a[i] = b[i];
}
void sort(int low, int high) {
int mid;
if(low < high) {
mid = (low + high) / 2;
sort(low, mid);
sort(mid+1, high);
merging(low, mid, high);
} else {
return;
}
}
int main() {
int i;
printf("List before sorting\n");
for(i = 0; i <= max; i++)
printf("%d ", a[i]);
sort(0, max);
printf("\nList after sorting\n");
for(i = 0; i <= max; i++)
printf("%d ", a[i]);
}
Post a Comment